A Quick Introduction to REBOL Scripting
Tutorials | ||
Learn REBOL (website) |
Revised: 12-Mar-2024
Original: 31-Jan-2003
Introduction
REBOL was designed for Internet communications. REBOL is an advanced interpreted language that lets you access and control the resources of the Internet with such ease that you might start thinking of the Internet as your own personal operating system. You're already familiar with how web browsers let you surf the Internet. With REBOL you script the Internet.
At REBOL headquarters we use REBOL for building manuals and documents (such as this one), generating our entire web site, automatically uploading pages and images to the net, sending email notices, processing credit cards, sharing files remotely, tracking customer orders, instant messaging, holding electronic meetings, auto-updating software, running our helpdesk, managing our bug database, removing junk mail, backing up servers, crawling the web, filtering ads from incoming web pages, and - most importantly - building REBOL across 42 unique systems.
Each day we use literally hundreds of REBOL scripts across dozens of machines to our great advantage. We need only one language for everything. Like the Wizard of Oz, REBOL amplifies the efforts of our tiny team to look like the work of hundreds.
This tutorial provides a concise introduction to using REBOL. If you've been procrastinating reading the 720 page REBOL manual, we understand. Who has time to read it all? With that in mind, the goal of this document is to give you a quick overview of REBOL in about 20 minutes and to provide links to where you can find out more.
A World of Datatypes
Languages are built from a basic set of datatypes that you use to construct everything else. Integer numbers and strings are examples of datatypes. Most languages have about five or six datatypes. By contrast, REBOL has 45 datatypes. The result is that for many tasks you can focus on what your program needs to do, rather than on how to do it.
For example, all languages let you add numbers:
101 + 10
However, in REBOL you can just as easily add times, dates, dollars, tuples, and coordinate pairs. Here are some examples followed by their results:
10:55 + 0:10 11:05 1-Jan-2001 + 100 11-Apr-2001 $10.55 + $100 $110.55 100.30.5 + 100.5.5 200.35.10 100x200 + 100x40 200x240
Note that you don't need any include files, import statements, or function declarations to use these datatypes. They are a fundamental part of the language.
Also note that you use the same notation for adding each of the types. Most functions work on multiple datatypes. They are polymorphic; they work with more than one datatype. The result? You become more productive, but without adding complexity to your program. Simple things should be simple.
Here is another example that searches the first string for an occurance of the second string:
find "World Wide Reb" "wide"
The same search operation works for many other datatypes such as email addresses, URLs, files, markup tags, issues (serial numbers), binary, and blocks:
find user@widetire.dom "wide" widetire.dom find http://data.rebol.com/wide/range.html "wide" wide/range.html find %/c/files/wide.txt "wide" %wide.txt find #ABC-WIDE-02120 "wide" #WIDE-02120 find #{746865207769646520776F726C64} "wide" #{7769646520776F726C64} find [123 10:30 "wide" 1-Jan-2001] "wide" ["wide" 1-Jan-2001]
Curious about the other 35 datatypes of REBOL? Type this line at the console to list them all:
help datatype!
Or take a look at the Values Appendix of the REBOL guide.
Integrated Networking
As the scripting language of the "Internet operating system", you'll need to be able to access Internet protocols with the same ease as you would files. REBOL has 14 standard protocols built-in. Again, there's no need for include files or function declarations. You just use them.
For example, to read and display a text file from your disk you would write: (Note that the percent sign indicates a file name in REBOL. It is required to prevent the filename from being interpreted as a REBOL word.)
print read %datafile.txt
You can just as easily read text from the network using a variety of protocols. The line below each example tells what it does:
read http://data.rebol.com/index.html (reads the HTML page from the web) read ftp://ftp.rebol.com/test.txt (downloads the file using FTP) read pop://fred:pass@example.com (reads all email messages, but does not delete them) read nntp://news.example.com/alt.test (reads all postings from a newsgroup) read whois://example.com@rs.internic.net (gets info about an internet domain) read finger://username@example.com (returns info about a user account) read daytime://everest.cclabs.missouri.edu (reads the time from a server) read dns:// (returns your local host name)
To try any of these out for yourself, put a print in front of the read and provide the valid URL info. Note that if you use a firewall, you may need to configure your REBOL proxy settings in order to see the results.
Of course, these examples are only the start. Many other features are available. For instance, to read an image from a web site and save it to a disk file:
write/binary %link.jpg read/binary http://data.rebol.com/graphics/link.jpg
The write function is used with a /binary refinement to indicate that this is a binary file, not a text file.
If you want to send a text file as the contents of an email, you would write:
send fred@example.com read %message.txt
But, you could just as easily send a web page with:
send fred@example.com read http://data.rebol.com
Of course, if all you want to do is send a text message, you can write:
send fred@example.com "Hello Fred!"
To write a line that reads an image file and uploads it to an Internet server, you can write:
write/binary ftp://user:pass@example.com/pub/link.jpg read/binary %link.jpg
Notice that you must provide the username and password as part of the URL.
Do you want to upload an entire directory of files? This script does the job:
site: ftp://user:pass@example.com/pub/ foreach file read %thefiles/ [ write/binary site/:file read/binary file ]
Notice there is another refinement being used on the site word. When a refinement is used on a URL, it combines the URL with the file name that is provided. The colon in front of file says "get the filename". Add a print to the loop to see the result:
print site/:file
Perhaps you want to upload only the JPEG files from that directory. Check the file names using find as was shown in the previous section:
foreach file read %thefiles/ [ if find file ".jpg" [ write/binary site/:file read/binary file ] ]
To upload all the GIF and JPEG files you can write:
foreach file read %thefiles/ [ if any [ find file ".jpg" find file ".gif" ] [ write/binary site/:file read/binary file ] ]
Now, let's say you want to upload only the files that have changed in the last two days:
date: now - 2 foreach file read %thefiles/ [ if (modified? file) > date [ write/binary site/:file read/binary file ] ]
The date variable is set by getting the current date and time, then subtracting two days from it. This is compared to the date on the file, which is obtained with the modified? function.
You could just as easily have written the loop to transfer files that were smaller than a certain size replacing the comparison line with:
if (size? file) < 100000 [
Or, you could avoid subdirectories with:
if not dir? file [
These functions also work with URLs. You can check the remote file size with:
print size? ftp://user:pass@example.com/pub/image.jpg
and you can check the file modification date with:
print modified? ftp://user:pass@example.com/pub/image.jpg
This level of Internet integration allows you to easily use network resources throughout your programs. For example, in REBOL/View if you want to create a window that uses an image file from the Internet, you write:
view layout [ image http://data.rebol.com/graphics/link.jpg ]
This would result in a window that shows the image:
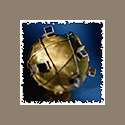
If you want to change the color of the window and make the image into a button that browses a web site, here is an expanded example:
view layout [ backcolor white image http://data.rebol.com/graphics/link.jpg [ browse http://data.rebol.com ] ]
It would look like:
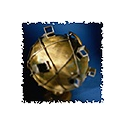
Similarly, you could add any of the previous network functions to this script. For instance, the button could FTP upload or download files, pick up email, send email, check a newsgroup, get info about a user, and anything else.
Finally, to show the full integration of REBOL with the Internet, you can run other REBOL programs directly from the net. They can be run from any of the protocols above that return a valid script. For example, here is what it takes to run a script from a web site:
do http://data.rebol.com/view/demos/gel.r
The do function downloads script and runs it. To run it with FTP you can write:
do ftp://www.rebol.com/pub/gel.r
You now have a better idea of how REBOL "scripts the Internet". As a final example, we combine the image button with with the do example.
view layout [ backcolor white image http://data.rebol.com/graphics/link.jpg [ do http://data.rebol.com/view/demos/gel.r ] ]
There you have it.
To learn more about networking, read the Networking chapter of the REBOL guide.
Creating Script Files
REBOL files can be used for code, data, or a combination of both. The files can be created using any text editor, or they can be created as the result of other programs. For instance, our company uses REBOL files to hold many of the databases that we use every day.
Every REBOL file begins with a header that identifies its contents, author, version, history, and a variety of other optional fields. This information is very useful, and we encourage you to provide it with your scripts. It helps with knowing:
- What the script does.
- Who wrote it.
- What version it is.
- When was it last modified.
- Who owns it.
- What filename should be used for it.
The script headers are always written in REBOL itself. They are meta-data that is readable by REBOL. This allows REBOL-based search engines and archive services to easily build useful indices of files. In addition, smart text editors and source version software can automatically track revisions.
Here is an example minimal header that you can use for any of the scripts that were shown above:
REBOL [ Title: "Short Title Here" Date: 10-July-2001 Author: "Your Name" Version: 1.0.0 ]
Notice that the date and version are not in quotes. They are written as REBOL datatypes. The first is a date, and the second is a tuple (note that it must contain at least three parts).
Taking the final example from the previous section, here is a complete example script file:
REBOL [ Title: "Gel Button" Date: 10-July-2001 Author: "Carl Sassenrath" Version: 1.0.0 ] view layout [ backcolor white image http://data.rebol.com/graphics/link.jpg [ do http://data.rebol.com/view/demos/gel.r ] ]
To find out more about REBOL scripts and their headers, see the Scripts chapter of the REBOL Guide.